Input/Output
Input is used to take information from the person using the program. The TextBox component is used to get input from a user. On your form put 2 TextBoxes, one named txtNum1, the other named txtNum2. Put a label with the Name property set to lblAnswer. All three of these components should have a blank Text property. Now put a Button on the form with the Name property btnCalc and the Text property set to Calculate. We are going to add code to the btnCalc_click procedure that will add the two numbers together. The code should look like this:
Private Sub btnCalc_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click lblAnswer.Text = txtNum1.Text + txtNum2.Text End Sub |
If you compiled that then you should be able to enter a number in each TextBox, push the Button and the sum of the two numbers should popup. Anything other than numeric data in those TextBoxes will cause an error. We’ll get into how to prevent that later on. With most programs, you will need to specify what goes in the TextBoxes, we do this by Labels before each TextBox giving instructions like Enter a Number, or something like that. When the Labels don’t change during runtime, we can just leave the default Name.
Say you wanted to put The answer is and then the answer inside the same Label. To do this we will need to concatanate the string with the component or variable.
Private Sub btnCalc_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click lblAnswer.Text = “The answer is ” & txtNum1.Text + txtNum2.Text End Sub |
We can also have the starting text inside the Label to be The answer is, then just add onto that. To do that we will need to conconcatenate what’s already inside the Text property. To do this we use &=
Private Sub btnCalc_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click lblAnswer.Text &= txtNum1.Text + txtNum2.Text End Sub |
Closing a Program
When closing a program, you usually have an X in the upper right hand corner of the screen, or a menu item that says Exit. Unless you change the ControlBox property on the form to False, the X will always be there. To add a menu to the form, we add a MenuStrip to the form then we can add which menus we want to add, and what appears in those menus. The naming convention for the menu strip varies a bit from other components, say we have a File menu and under it we have Exit. File would be named mnuFile and the exit under the file would be named mnuFileExit. In case you haven’t figured it out, the main menus would go horizontal and the submenus would go underneith the selected menu item, let’s add a File/Exit menu. When you’re done it should look like this:
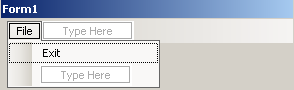
If you run the program, it will allow you to select File, you’ll see Exit, but exit doesn’t do anything. Double click on the Exit menu item to bring up the mnuFileExit_Click subprocedure. There are two ways to write the code for exiting a program, one way is to use simply type Exit. This way will abruptly end the program and not clean itself up, much like hitting CTRL ALT DEL and ending a program that way. The proper way to end a program is with Me.Close(). Here’s how it would look:
Private Sub mnuFileExit_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles mnuFileExit.Click Me.Close() End Sub |
So far, you’ve learned some of the simplest commands in the Visual BASIC. Keep in mind that the code, while I may show it in one place, would probably work in other subprocedures.
Compared to other programming languages, the basics to Visual BASIC are very simple. The next obvious step is to delve deeper into the programming. You must have a solid foundation in this first, however, because if you don’t you most likely will be lost.